Basic Structure of a JavaScript Program
A JavaScript program is made up of one or more scripts. A script is a sequence of statements that tell the computer what to do. Here are some of the scripts commonly used in JavaScript;
1. console.log() and alert(): console.log is used to display output in the browser's console whereas alert is a built-in function that displays a pop-up box with a specified message
console.log("Hello world!");
//This code will display the text "Hello, world!" in the browser's console
alert("Hello world!");
//This code will display a pop-up box with the text "Hello, world!"
2. Variables: In JavaScript, a variable is a named container that holds a value. You can declare a variable using the var, let, or const keyword, followed by the variable name. For example:
// Using let (used when a variable is expected to change) where; age is the variable name
let age = 25;
// Using const (constant value) where; country is the variable name
const country = "USA";
3. Data Types: JavaScript supports various data types:
a. String: A sequence of characters, represented by enclosing the characters in quotes (single or double). Examples: "Hello", 'World', '20'. To find the length of a string, use the built-in length property:
let alphabets = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
let numberOfAlphabets = alphabets.length;
console.log(numberOfAlphabets)
//it will display 26 on th browser console.
String Methods: These are methods that allow you to perform operations such as searching, modifying, and analyzing strings.
String slice(): slice() extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: start position, and end position (end not included).
slice(start, end): This method extracts a part of a string by specifying the start and end index of the substring to be extracted. The start index is inclusive and the end index is exclusive, meaning that the substring will include all characters from the start index up to, but not including, the end index. For example
// Declare a variable 'str' and assign it the string value "Hello World"
let str = "Hello World";
// Use the 'slice' method on the string 'str'.
// The 'slice' method extracts a section of the string and returns it as a new string.
// The 'slice' method takes two arguments: the start index and the end index (not inclusive).
// In this case, slice(0, 5) means: start at index 0 (the first character) and end at index 5 (not including the character at index 5).
console.log(str.slice(0, 5)); // This will output the substring "Hello"
How the it was done
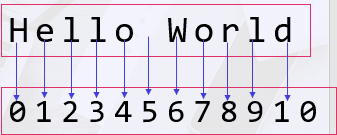
substring(start, end): This method works similarly to slice(), but it does not accept negative indexes. It also corrects the order of start and end if it's passed in reverse order, which means substring(end, start) will give you the same result as substring(start, end). mc
replace(): method is used to replace all occurrences of a specified value in a string with a new value. The method takes two arguments: the first argument is the value to be replaced, and the second argument is the new value that will replace the old value. The method returns a new string with the replaced values, and the original string remains unchanged, for example;
let str = “Welcome to Microsoft";
let newStr = str.replace(“Microsoft", “Desishub");
console.log(newStr); // “Welcome to Desishub"
The replace() method by default it replaces only the first match. If you want to replace all matches, use a regular expression with the /g flag set. See examples below.
str.replace(/World/g, "JavaScript"); console.log(newStr); // "Hello JavaScript, today
the JavaScript is growing fast" ``` In this example, the regular expression /World/g
is used to match the substring "World". The "g" flag is used to specify that the
replacement should happen globally, which means all occurrences of the substring
in the string will be replaced.{" "}
**Converting to Upper and Lower Case:** In JavaScript, you can convert a string to uppercase by using the toUpperCase() method and to lowercase by using the toLowerCase() method.
```js copy
let str = "Hello World";
let upperStr = str.toUpperCase(); // "HELLO WORLD"
let lowerStr = str.toLowerCase(); // "hello world"
Joining strings Using concat(): The concat() method in JavaScript is used to join two or more strings together. The method can take any number of arguments and it returns a new string that is the concatenation of all the given strings.
let str1 = "Hello";
let str2 = "World";
let newStr = str1.concat(" ", str2); // "Hello World"
You can also use the + operator to concatenate strings in JavaScript. The + operator is more commonly used for string concatenation.
let str1 = "Hello";
let str2 = "World";
let newStr = str1 + " " + str2; // "Hello World"
trim(): The trim() method removes whitespace from both sides of a string
let text1 = " Hello World! ";
let text2 = text1.trim();
Note: ECMAScript 2019 added the String methods trimStart() and trimStart() to JavaScript. The trimStart() method works like trim(), but removes whitespace only from the start of a string. The trimEnd() method works like trim(), but removes whitespace only from the end of a string.
charAt(position): This method returns the character at the specified index (position) in a string. The first character has an index of 0, the second has an index of 1, and so on. If the specified index is out of range, the method returns an empty string.
let str = "Hello World";
let char = str.charAt(0); // "H"
charCodeAt(position): This method returns the Unicode of the character at the specified index in a string. The Unicode code is a unique number that represents each character in a string. The method returns a UTF-16 code (an integer between 0 and 65535).
let str = "Hello World";
let code = str.charCodeAt(0); // 72
Property access [ ]: This is an alternative way of accessing individual characters in a string. You can use square brackets [] to access a character at a specific index.
let str = "Hello World";
let char = str[0]; // "H"
split() method: The split() method is used to split a string into an array of substrings based on a specified separator. By default, it splits the string into an array of characters if no separator is provided.
let str = "Hello World";
let charArray = str.split(""); // ["H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d"]
//Using Space as a separator
let str = "Hello World";
let words = str.split(" "); // ["Hello", "World"]
//Using Comma as a separator
let str = "apple,banana,orange";
let fruits = str.split(","); // ["apple", "banana", "orange"]
//Using limit as a separator
let str = "apple,banana,orange,mango";
let fruits = str.split(",", 3); // ["apple", "banana", "orange,mango"]
As you can see, the split() method takes a separator as its argument, and it can be a string, regular expression, or any other value that is used to split the string into an array of substrings. The separator is not included in the returned array, and if no separator is provided, it splits the string into an array of characters. You can also specify a limit to the number of times the separator can be used to split the string, if the limit is reached, the rest of the string will be considered as a single element of the array.
b. Number: A numerical value. Examples: 123, 3.14.
Number Methods:
toString(): Returns a number as a string
toExponential(): Returns a number written in exponential notation
toFixed(): Returns a number written with a number of decimals
toPrecision(): Returns a number written with a specified length
ValueOf(): Returns a number as a number
c. Boolean: A value that can be either true or false. Used for taking Decisions.
d. Undefined: : A value that is assigned to a variable that has not been given a value yet.
e. Null: A special value that represents an absence of value.
f. Array: In JavaScript, arrays can be a collection of elements of any type. This means that you can create an array with elements of type String, Boolean, Number, Objects, and even other Arrays. A pair of square brackets [] represents an array in JavaScript. All the elements in the array are comma(,) separated. The position of an element in the array is known as its index. In JavaScript, the array index starts with 0, and it increases by one with each element.
Object: Objects store data in key-value pairs.
//String
let name = 'Alice';
//Number
let age = 30;
//Boolean
let isStudent = true;
//Array
let hobbies = ['reading', 'traveling', 'coding'];
//Object
let person = {
firstName: 'John',
lastName: 'Doe',
age: 40
};
4. Operetors: operators allow you to manipulate data, make comparisons, and control the flow of your code. Here is a more detailed look at the various types of operators in JavaScript.
a. Arithmetic Operators: Arithmetic operators are used to perform basic mathematical operations.
Addition (+): used in addition instances.
const sum = 2 + 3;
console.log(sum)
//It will display 5 in the browser's console.
Subtraction (-): Subtracts one number from another.
const difference = 5 - 3;
console.log(difference)
//It will display 2 in the browser's console.
Multiplication (*): Multiplies two numbers.
const product = 2 * 3;
console.log(product)
//It will display 6 in the browser's console.
Division (/): Divides one number by another.
let quotient = 6 / 3;
console.log(quotient)
//It will display 2 in the browser's console.
Modulus (%): Returns the remainder of a division.
let remainder = 7 % 3;
console.log(remainder)
//It will display 1 in the browser's console.
Increment (++): Increases a number by one.
let x = 5;
x++;
console.log(X)
//It will display 6 in the browser's console.
Decrement (--): Decreases a number by one.
let Y = 5;
Y--;
console.log(Y)
//It will display 4 in the browser's console.
b. Assignment Operators: Assignment operators are used to assign values to variables.
Assignment (=): Assigns a value to a variable.
let x = 10;
console.log(X)
c. Comparison Operators: Comparison operators compare two values and return a boolean result ('true' or 'false').
Equal (==): Compares two values for equality (type conversion is performed).
let result = (5 == '5'); // true
console.log(result)
//It will display true in the browser's console.
**Strict Equal (===): ** Compares two values for equality without type conversion.
let result = (5 === '5'); // true
console.log(result)
//It will display false in the browser's console.
Not Equal (!=): Compares two values for inequality (type conversion is performed).
const result = (5 != '5');
console.log(result)
//It will display false in the browser's console.
Strict Not Equal (!==): Compares two values for inequality without type conversion.
let result = (5 !== '5');
console.log(result)
//It will display true in the browser's console.
Greater Than (>): Checks if the left value is greater than the right value.
const result = (10 > 5);
console.log(result)
//It will display true in the browser's console.
Less Than: Checks if the left value is less than the right value.
let result = (10 < 5);
console.log(result)
//It will display false in the browser's console.
Greater Than or Equal (>=): Checks if the left value is greater than or equal to the right value.
let result = (10 >= 10);
console.log(result)
//It will display true in the browser's console.
Less Than or Equal (<=
): Checks if the left value is less than or equal to the right value.
let result = (10 <= 10);
console.log(result)
//It will display true in the browser's console.
c. Logical Operators: Logical operators are used to combine multiple boolean expressions.
AND (&&): Returns true if both operands are true.
const result = (5 > 3 && 10 > 5); // true
console.log(result)
//It will display true in the browser's console.
OR (||): Returns true if at least one of the operands is true.
const result = (5 > 3 || 10 < 5); // true
console.log(result)
//It will display true in the browser's console.
NOT (!): Returns true if the operand is false.
let result = !(5 > 3);
console.log(result)
//It will display false in the browser's console.
d. Conditional operator (? :): The conditional operator, also known as the ternary operator, is a shorthand way of writing an if statement. It is used with the ? and : symbols. For example:
let x = 10;
let y = 20;
let max = (x > y) ? x : y; // max is 20
This code is equivalent to the following if statement:
let max;
if (x > y) {
max = x;
} else {
max = y;
}
5. Control Structures: Control structures are used to control the flow of a program, based on certain conditions.
Conditional Statements: Control the flow of your code using conditional statements.
let time = 20;
if (time < 12) {
console.log('Good morning!');
} else if (time < 18) {
console.log('Good afternoon!');
} else {
console.log('Good evening!');
}