Javascript Functions
Javascript Function types
Function declarations:
Function declarations are created using the function keyword, followed by the function name and a set of parentheses that may include parameters. The function body is placed between curly braces. Function declarations are hoisted, which means that they are available for use throughout your code, even before they are defined. Here is an example of a function declaration:
function sayHello(name) {
console.log("Hello, " + name + "!");
}
Function expressions:
Function expressions are created by assigning a function to a variable. They are not hoisted, which means that they are not available for use until after they are defined. Here is an example of a function expression:
var sayHello = function (name) {
console.log("Hello, " + name + "!");
};
Arrow functions:
Arrow functions are a modern way to write functions in JavaScript. They are created using the => syntax and are concise and easy to read. Arrow functions do not have their own this value and cannot be used as constructors. Here is an example of an arrow function:
const sayHello = (name) => {
console.log("Hello, " + name + "!");
};
Calling(Invoking Functions): To call a function in JavaScript, you simply need to use its name followed by a set of parentheses that may include arguments. For example:
function sayHello(name) {
console.log("Hello, " + name + "!");
}
//Calling the function
sayHello("John");
//It will display Hello John! on the browser console
function parameters: are the names listed in the function definition and are used to receive input values (arguments) when the function is called. The function can then use these parameters to perform its task. For example:
function add(a, b) {
return a + b;
}
Sum = add(1, 2);
console.log(sum);
//It will the result after adding 1 and 2 which 3.
In this example, the add function accepts two parameters, a and b, and returns their sum. When the function is called with the arguments 1 and 2, it returns the value 3.
A 'return statement' is used within a function to specify the value that the function should output when it is called. The return statement ends the function's execution and specifies the value that should be returned to the caller. If a function does not have a return statement, it will return the special value undefined.
ANATOMY OF A FUNCTION:
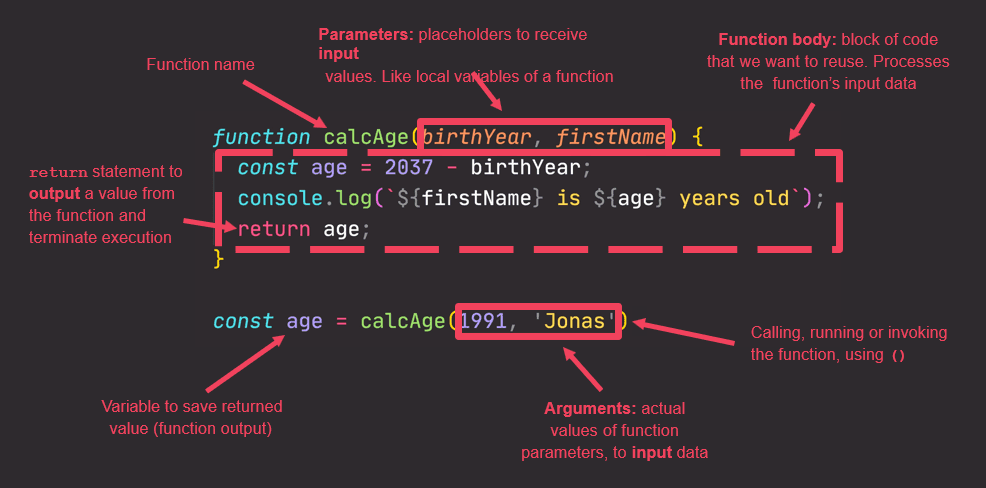